Nos clients nous font confiance
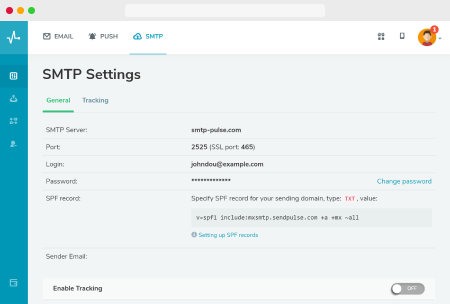
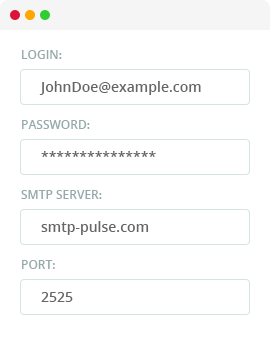
Envoi de messages via la passerelle
Les courriels transactionnels sont des messages automatiques envoyés en réponse aux actions d’un abonné. Abonnement ou confirmation de réservation, mises à jour de commande, notifications - ce sont tous des exemples de courriels transactionnels.
Pour envoyer des courriels transactionnels via SMTP, saisissez une adresse de serveur, un port, un identifiant et le mot de passe de votre compte SendPulse dans votre application.
<?php
use Sendpulse\RestApi\ApiClient;
use Sendpulse\RestApi\Storage\FileStorage;
$smtpSendMailResult = (new ApiClient('MY_API_ID', 'MY_API_SECRET', new FileStorage()))->post('smtp/emails', [
'email' => [
'html' => base64_encode('<p>Hello!</p>'),
'text' => 'text',
'subject' => 'Mail subject',
'from' => ['name' => 'API package test', 'email' => 'from@test.com'],
'to' => [['name' => 'to', 'email' => 'to@test.com']],
'attachments_binary' => [
'attach_image.jpg' => base64_encode(file_get_contents('../storage/attach_image.jpg'))
]
]]);
var_dump($smtpSendMailResult);
?>
sample.php hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-php
# SendPulse's Ruby Library: https://github.com/sendpulse/sendpulse-rest-api-ruby
require './api/sendpulse_api'
sendpulse_api = SendpulseApi.new(API_CLIENT_ID, API_CLIENT_SECRET, API_PROTOCOL, API_TOKEN)
email = {
html: '<p>Your email content goes here<p>',
text: 'Your email text version goes here',
subject: 'Testing SendPulse API',
from: { name: 'Your Sender Name', email: 'your-sender-email@gmail.com' },
to: [
{
name: "Subscriber's name",
email: 'subscriber@gmail.com'
}
]
}
sendpulse_api.smtp_send_mail(email)
sample.rb hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-ruby
# SendPulse's Python Library: https://github.com/sendpulse/sendpulse-rest-api-python
from pysendpulse import PySendPulse
if __name__ == "__main__":
TOKEN_STORAGE = 'memcached'
SPApiProxy = PySendPulse(REST_API_ID, REST_API_SECRET, TOKEN_STORAGE)
email = {
'subject': 'This is the test task from REST API',
'html': '<p>This is a test task from https://sendpulse.com/api REST API!<p>',
'text': 'This is a test task from https://sendpulse.com/api REST API!',
'from': {'name': 'John Doe', 'email': 'john.doe@example.com'},
'to': [
{'name': 'Jane Roe', 'email': 'jane.roe@example.com'}
]
}
SPApiProxy.smtp_send_mail(email)
sample.py hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-python
// SendPulse's Java Library https://github.com/sendpulse/sendpulse-rest-api-java
import com.sendpulse.restapi.Sendpulse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
public class Example {
public static void main(String[] args) {
Sendpulse sendpulse = new Sendpulse(API_CLIENT_ID, API_CLIENT_SECRET);
Map<String, Object> from = new HashMap<String, Object>();
from.put("name", "Your Sender Name");
from.put("email", "your-sender-email@gmail.com");
ArrayList<Map> to = new ArrayList<Map>();
Map<String, Object> elementto = new HashMap<String, Object>();
elementto.put("name", "Subscriber's name");
elementto.put("email", "subscriber@gmail.com");
to.add(elementto);
Map<String, Object> emaildata = new HashMap<String, Object>();
emaildata.put("html","Your email content goes here");
emaildata.put("text","Your email text version goes here");
emaildata.put("subject","Testing SendPulse API");
emaildata.put("from",from);
emaildata.put("to",to);
Map<String, Object> result = (Map<String, Object>) sendpulse.smtpSendMail(emaildata);
System.out.println("Result: " + result);
}
}
sample.java hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-java
// SendPulse's Node.JS Library: https://github.com/sendpulse/sendpulse-rest-api-node.js
var sendpulse = require("./api/sendpulse.js");
sendpulse.init(API_USER_ID, API_SECRET, TOKEN_STORAGE);
var email = {
"html" : "<p>Your email content goes here</p>",
"text" : "Your email text version goes here",
"subject" : "Testing SendPulse API",
"from" : {
"name" : "Your Sender Name",
"email" : "your-sender-email@gmail.com"
},
"to" : [ {
"name" : "Subscriber's name",
"email" : "subscriber@gmail.com"
} ]
};
var answerGetter = function answerGetter(data){
console.log(data);
}
sendpulse.smtpSendMail(answerGetter, email);
sample.js hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-//www.spcdn.org/node.js
#import "Sendpulse.h" // SendPulse's Obj-C Library https://github.com/sendpulse/sendpulse-rest-api-objective-c
- (void)viewDidLoad {
[super viewDidLoad];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(doSomethingWithTheData:) name:@"SendPulseNotification" object:nil];
Sendpulse* sendpulse = [[Sendpulse alloc] initWithUserIdandSecret:userId :secret];
NSDictionary *from = [NSDictionary dictionaryWithObjectsAndKeys:@"Your Sender Name", @"name", @"your-sender-email@gmail.com", @"email", nil];
NSMutableArray* to = [[NSMutableArray alloc] initWithObjects:[NSDictionary dictionaryWithObjectsAndKeys:@"Subscriber's name", @"name", @"subscriber@gmail.com", @"email", nil], nil];
NSMutableDictionary *emaildata = [NSMutableDictionary dictionaryWithObjectsAndKeys:@"<b>Your email content goes here</b>", @"html", @"Your email text version goes here", @"text",@"Testing SendPulse API",@"subject",from,@"from",to,@"to", nil];
[sendpulse smtpSendMail:emaildata];
}
- (void)doSomethingWithTheData:(NSNotification *)notification {
NSMutableDictionary * result = [[notification userInfo] objectForKey:@"SendPulseData"];
NSLog(@"Result: %@", result);
}
sample.m hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-objective-cAPI SMTP
L'API SMTP vous permet d'envoyer des courriels transactionnels aux abonnés à partir de votre site Internet, de votre CRM ou d'autres applications Internet.
Rapports d'efficacité du courriel
Mesurez régulièrement les statistiques de vos courriels pour améliorer vos performances de marketing par courriel et votre retour sur investissement. Tous les rapports SendPulse - sur les taux d'ouverture et de clics, l'emplacement de la boîte de réception, les taux de livraison et les erreurs et les plaintes pour courriel indésirable - peuvent être téléchargés.
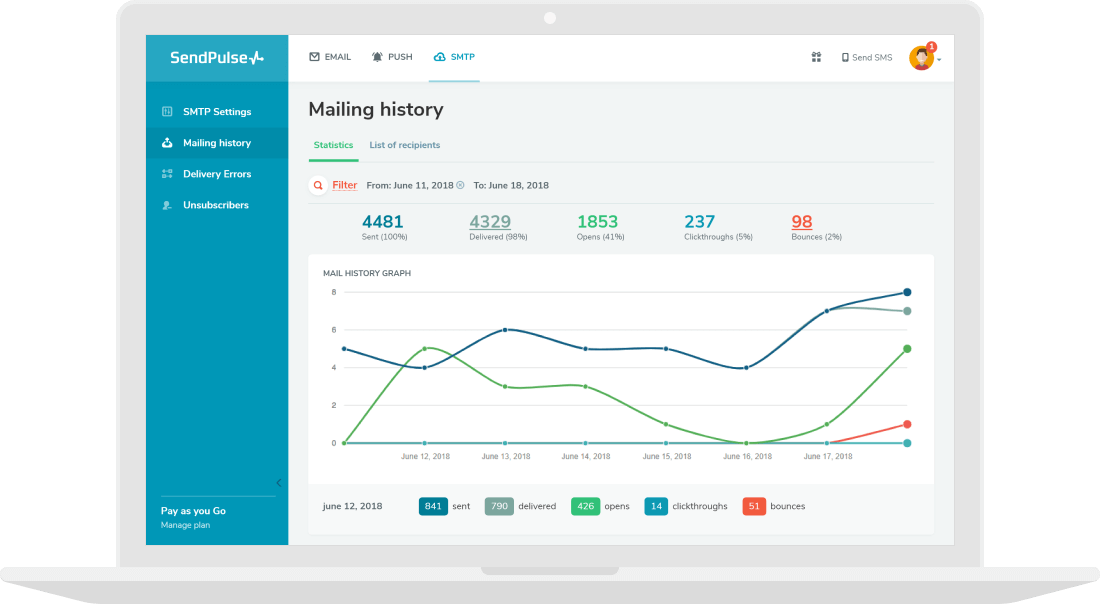
Outils faciles à utiliser pour envoyer des courriels transactionnels
ADRESSES IP DÉDIÉES
Une adresse dédiée contribue à améliorer la réputation de l'expéditeur et empêche votre IP d'être inscrite sur la liste noire.
AUTHENTIFICATION SPF ET DKIM
Les enregistrements SPF et DKIM empêchent les courriels transactionnels d’atterrir dans un dossier de courrier indésirable.
LISTE DES CONTACTS DÉSABONNÉS
Vos courriels ne seront pas envoyés à des contacts désabonnés, même s'ils figurent sur une liste de diffusion.
OUVERTURE ET SUIVI DE CLIC
Des rapports détaillés aident à évaluer et à améliorer l'efficacité de votre marketing par courriel.
DOMAINE DE SUIVI PERSONNALISÉ
Envoyez des courriels transactionnels sans aucune mention de SendPulse dans l'en-tête et le pied de page.
CROCHETS INTERNET
Recevez des informations sur l'état des courriels sur votre système : livrés, non livrés, ouverts et cliqués.
ou